📜 [專欄新文章] Gas Efficient Card Drawing in Solidity
✍️ Ping Chen
📥 歡迎投稿: https://medium.com/taipei-ethereum-meetup #徵技術分享文 #使用心得 #教學文 #medium
Assign random numbers as the index of newly minted NFTs
Scenario
The fun of generative art NFT projects depends on randomness. The industry standard is “blind box”, where both the images’ serial number and the NFTs’ index are predetermined but will be shifted randomly when the selling period ends. (They call it “reveal”) This approach effectively solves the randomness issue. However, it also requires buyers to wait until the campaign terminates. What if buyers want to know the exact card right away? We’ll need a reliable onchain card drawing solution.
The creator of Astrogator🐊 isn’t a fan of blind boxes; instead, it thinks unpacking cards right after purchase is more interesting.
Spec
When initializing this NFT contract, the creator will determine the total supply of it. And there will be an iterable function that is randomly picking a number from the remaining pool. The number must be in range and must not collide with any existing ones.
Our top priority is accessibility/gas efficiency. Given that gas cost on Ethereum is damn high nowadays, we need an elegant algorithm to control gas expanse at an acceptable range.
Achieving robust randomness isn’t the primary goal here. We assume there’s no strong financial incentive to cheat, so the RNG isn’t specified. Implementers can bring their own source of randomness that they think is good enough.
Implementation
Overview
The implementation is pretty short and straightforward. Imagine there’s an array that contains all remaining(unsold) cards. When drawIndex() is called, it generates a (uniform) random seed to draw a card from the array, shortens the array, and returns the selected card.
Algorithm
Drawing X cards from a deck with the same X amount of cards is equal to shuffling the deck and dealing them sequentially. It’s not a surprise that our algorithm is similar to random shuffling, and the only difference is turning that classic algo into an interactive version.
A typical random shuffle looks like this: for an array with N elements, you randomly pick a number i in (0,N), swap array[0] and array[i], then choose another number i in (1,N), swap array[1] and array[i], and so on. Eventually, you’ll get a mathematically random array in O(N) time.
So, the concept of our random card dealing is the same. When a user mints a new card, the smart contract picks a number in the array as NFT index, then grabs a number from the tail to fill the vacancy, in order to keep the array continuous.
Tweak
Furthermore, as long as the space of the NFT index is known, we don’t need to declare/initialize an array(which is super gas-intensive). Instead, assume there’s such an array that the n-th element is n, we don’t actually initialize it (so it is an array only contains “0”) until the rule is broken.
For the convenience of explanation, let’s call that mapping cache. If cache[i] is empty, it should be interpreted as i instead of 0. On the other hand, when a number is chosen and used, we’ll need to fill it up with another unused number. An intuitive method is to pick a number from the end of the array, since the length of the array is going to decrease by 1.
By doing so, the gas cost in the worst-case scenario is bound to be constant.
Performance and limitation
Comparing with the normal ascending index NFT minting, our random NFT implementation requires two extra SSTORE and one extra SLOAD, which cost 12600 ~ 27600 (5000+20000+2600) excess gas per token minted.
Theoretically, any instantly generated onchain random number is vulnerable. We can restrict contract interaction to mitigate risk. The mitigation is far from perfect, but it is the tradeoff that we have to accept.
ping.eth
Gas Efficient Card Drawing in Solidity was originally published in Taipei Ethereum Meetup on Medium, where people are continuing the conversation by highlighting and responding to this story.
👏 歡迎轉載分享鼓掌
同時也有9部Youtube影片,追蹤數超過12萬的網紅prasertcbs,也在其Youtube影片中提到,? เทคนิคต่าง ๆ ที่ใช้ในคลิป 1. การแปลง Range ให้เป็น Table 2. การใช้ฟังก์ชัน ROWS() เพื่อนับจำนวนแถวใน Table 3. การใช้ฟังก์ชัน INDEX() เพื่อถึงข้อมูลใ...
「array index」的推薦目錄:
- 關於array index 在 Taipei Ethereum Meetup Facebook 的最讚貼文
- 關於array index 在 Taipei Ethereum Meetup Facebook 的最讚貼文
- 關於array index 在 BorntoDev Facebook 的精選貼文
- 關於array index 在 prasertcbs Youtube 的精選貼文
- 關於array index 在 prasertcbs Youtube 的最佳貼文
- 關於array index 在 prasertcbs Youtube 的最佳貼文
- 關於array index 在 JavaScript Array indexOf and lastIndexOf: Locating an ... 的評價
- 關於array index 在 Using Array.at(index) instead of Array[index] in JavaScript 的評價
- 關於array index 在 Laravel Array Validation: Set Messages with Position/Index 的評價
- 關於array index 在 For in array should be number index? #47578 - GitHub 的評價
array index 在 Taipei Ethereum Meetup Facebook 的最讚貼文
📜 [專欄新文章] Solidity Data Collision
✍️ Wias Liaw
📥 歡迎投稿: https://medium.com/taipei-ethereum-meetup #徵技術分享文 #使用心得 #教學文 #medium
這是一篇關於 Proxy Contract 和 delegatecall 的注意事項。
Delegatecall
當 A 合約對 B 合約執行 delegatecall 時,B 合約的函式會被執行,但是對 storage 的操作都會作用在 A 合約上。舉例如下:
但是假如多加了一個 other 欄位在 _value 之前,執行合約之後反而是 other 欄位被更改了。
Storage Layout
了解上面的合約之前要先了解 Solidity 怎麼儲存 State Variables。Solidity Storage 以 Slot 為單位,每個 Slot 可以儲存 32 bytes 的資訊,一個 Contract 擁有 2**256 個 Slot。上述可以寫成一個映射關係 mapping(uint256 => bytes32) slots。
Solidity 會從 Slot Index 為零開始分配給 State Variable。
除了 mapping 和 dynamically-sized array,其他的 State Variable 會從 index 為零的 slot 開始被分配。
沒有宣告確切大小的 Array 會以 Slot Index 計算出一個雜湊值並將其作為 Slot Index。透過計算 keccak256(slot) 可以得知 _arr[0] 被存在哪裡,如果要取得 _arr[1] 則將計算出來的雜湊加上 Array 的 index 即可。
Mapping 則是以 Slot Index 和 Key 計算出一個雜湊值並將其作為 Slot Index。透過計算 keccak256(key, slot) 可以得到 mapping(key => value) 被存在哪。
Storage Collision
回到 DelegateExample_v2 的合約,對 B 來說, add 最後儲存加法的 Slot Index 為零,所以使用 A 的 Storage 執行 B 的函式結果自然會儲存在 A 的 other 裡,其 Slot Index 為 0。
這個問題也發生在 Proxy Contract,Layout 如下,當有需要更改 _owner 的操作,就會順帶把 _implementation 也更改了。
|Proxy |Implementation ||--------------------------|-------------------------||address _implementation |address _owner | <= collision|... |mapping _balances || |uint256 _supply || |... |
OpenZeppelin 處理的手法也很簡單,就是將 _implementation 換地方擺。以特定字串的雜湊值作為 Slot Index,儲存 Implementation 的地址。
|Proxy |Implementation ||--------------------------|-------------------------||... |address _owner ||... |mapping _balances ||... |uint256 _supply ||... |... ||address _implementation | | <= specified|... | |
openzeppelin-contracts/ERC1967Upgrade.sol at 83644fdb6a9f75a652d2fe2d96cb26073a14f6f8 · OpenZeppelin/openzeppelin-contracts
hardhat-storage-layout
如何知道合約的 Storage Layout 呢?這邊推薦一個 Hardhat Plugin,按照文件就能得到合約的 Storage Layout。
Ethereum development environment for professionals by Nomic Labs
Reference
Understanding Ethereum Smart Contract Storage
Collisions of Solidity Storage Layouts
Proxy Upgrade Pattern - OpenZeppelin Docs
Solidity Data Collision was originally published in Taipei Ethereum Meetup on Medium, where people are continuing the conversation by highlighting and responding to this story.
👏 歡迎轉載分享鼓掌
array index 在 BorntoDev Facebook 的精選貼文
💡 สวัสดีจ้าเพื่อน ๆ วันนี้แอดจะพาเพื่อน ๆ มารู้จักกับ Operator จาก JavaScript ที่จะช่วยให้เพื่อน ๆ เข้าถึงข้อมูลใน Object ได้ง่ายมากขึ้น !!
.
🌈 และเจ้านี่คือ...Optional chaining (?.) นั่นเองจ้า จะเป็นยังไง มีรายละเอียด และวิธีการใช้งานยังไง ไปติดตามกันได้ในโพสต์นี้เลยจ้า ~~
.
✨ Optional chaining (?.) - เป็นตัวดำเนินการที่ทำให้เราสามารถอ่านค่าใน Object ที่ซ้อนกันหลาย ๆ ชั้นได้ง่ายมากขึ้น เขียนง่าย และทำให้โค้ดสั้นลงนั่นเอง
.
จริง ๆ แล้วมันก็เหมือนเราใช้ เครื่องหมายจุด (.) นั่นแหละ แต่ความพิเศษของมันก็คือถ้าในกรณีไม่มีค่าใน Object หรือ Function มันจะ Return เป็น Undefined แทน Error
.
👨💻 Syntax
.
obj.val?.prop
obj.val?.[expr]
obj.arr?.[index]
obj.func?.(args)
.
📑 วิธีการใช้งาน
.
❤️ ตัวอย่าง 1 : ใช้เข้าถึงข้อมูลใน Object
let customer = {
name: "Mew",
details: {
age: 19,
location: "Ladprao",
city: "bangkok"
}
};
let customerCity = customer.details?.city;
console.log(customerCity);
//output => bangkok
.
❤️ ตัวอย่าง 2 : ใช้กับ Nullish Coalescing
let customer = {
name: "Mew",
details: {
age: 19,
location: "Ladprao",
city: "bangkok"
}
};
const customerName = customer?.name ?? "Unknown customer name";
console.log(customerName); //output => Mew
.
❤️ ตัวอย่าง 3 : ใช้กับ Array
const obj1 = {
arr1:[45,25,14,7,1],
obj2: {
arr2:[15,112,9,10,11]
}
}
console.log(obj1?.obj2?.arr2[1]); // output => 112
console.log(obj1?.arr1[5]); // output => undefined
.
📑 Source : https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Optional_chaining
.
borntoDev - 🦖 สร้างการเรียนรู้ที่ดีสำหรับสายไอทีในทุกวัน
#javascript #optionalchaining #BorntoDev
array index 在 prasertcbs Youtube 的精選貼文
? เทคนิคต่าง ๆ ที่ใช้ในคลิป
1. การแปลง Range ให้เป็น Table
2. การใช้ฟังก์ชัน ROWS() เพื่อนับจำนวนแถวใน Table
3. การใช้ฟังก์ชัน INDEX() เพื่อถึงข้อมูลในแถวที่ต้องการ เช่น แถวสุดท้าย
4. การใช้ฟังก์ชัน INDEX() เพื่อส่งข้อมูลกลับมาหลาย ๆ คอลัมน์พร้อม ๆ กัน
ดาวน์โหลดไฟล์ Excel ที่ใช้ในคลิปได้ที่ ► https://bit.ly/3crgaMy
? เชิญสมัครเป็นสมาชิกของช่องนี้ได้ที่ ► https://www.youtube.com/subscription_center?add_user=prasertcbs
สอน Excel ► https://www.youtube.com/playlist?list=PLoTScYm9O0GEMj5LpqxaxWWnanc55Epnt
สอน Excel เบื้องต้น ► https://www.youtube.com/playlist?list=PLoTScYm9O0GEG_nWHhWmV0K2HsLlQ49qV
สอนการสร้างกราฟด้วย Excel ► https://www.youtube.com/playlist?list=PLoTScYm9O0GExxZ3nlVmleu0wvlhGfs3j
สอน PivotTable ► https://www.youtube.com/playlist?list=PLoTScYm9O0GFFdZwK6437TxMXYf7Hrd4I
สอน Excel Table ► https://www.youtube.com/playlist?list=PLoTScYm9O0GERViw9rwiISMWBv8rzT1j3
สอน Power Query ► https://www.youtube.com/playlist?list=PLoTScYm9O0GHrWoIfdwu9p8V2aNGzPauA
สอน Conditional formatting ► https://www.youtube.com/playlist?list=PLoTScYm9O0GGf0d7N6EfMrxiXZm3LukPV
สอน Excel Macro/VBA ► https://www.youtube.com/playlist?list=PLoTScYm9O0GHgpbmyNuXP39OUcb0BheaE
สอนเทคนิคการใช้งาน PowerPoint ► https://www.youtube.com/playlist?list=PLoTScYm9O0GEG5JELOjSGqigFN669d5IK
สอนเทคนิคการใช้งาน Word ► https://www.youtube.com/playlist?list=PLoTScYm9O0GG5QrQtl8hmVbg0o8fCCaJT
#prasertcbs_Excel #prasertcbs_ExcelForBusines #prasertcbs #prasertcbs_basic_excel #prasertcbs_chart

array index 在 prasertcbs Youtube 的最佳貼文
ดาวน์โหลดไฟล์ตัวอย่างได้ที่ https://goo.gl/rxG8dY
เชิญสมัครเป็นสมาชิกของช่องนี้ได้ที่ ► https://www.youtube.com/subscription_center?add_user=prasertcbs
สอน Excel ► https://www.youtube.com/playlist?list=PLoTScYm9O0GEMj5LpqxaxWWnanc55Epnt
สอน Excel เบื้องต้น ► https://www.youtube.com/playlist?list=PLoTScYm9O0GEG_nWHhWmV0K2HsLlQ49qV
สอนการสร้างกราฟด้วย Excel ► https://www.youtube.com/playlist?list=PLoTScYm9O0GExxZ3nlVmleu0wvlhGfs3j
สอน PivotTable ► https://www.youtube.com/playlist?list=PLoTScYm9O0GFFdZwK6437TxMXYf7Hrd4I
สอน Excel Table ► https://www.youtube.com/playlist?list=PLoTScYm9O0GERViw9rwiISMWBv8rzT1j3
สอน Power Query ► https://www.youtube.com/playlist?list=PLoTScYm9O0GHrWoIfdwu9p8V2aNGzPauA
สอน Excel Macro/VBA ► https://www.youtube.com/playlist?list=PLoTScYm9O0GHgpbmyNuXP39OUcb0BheaE
สอนเทคนิคการใช้งาน PowerPoint ► https://www.youtube.com/playlist?list=PLoTScYm9O0GEG5JELOjSGqigFN669d5IK
สอนเทคนิคการใช้งาน Word ► https://www.youtube.com/playlist?list=PLoTScYm9O0GG5QrQtl8hmVbg0o8fCCaJT
#prasertcbs_Excel #prasertcbs_ExcelForBusines #prasertcbs #prasertcbs_basic_excel #prasertcbs_chart
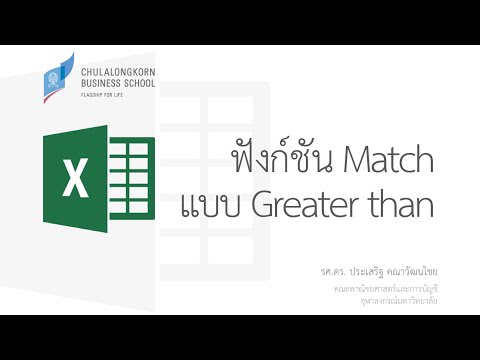
array index 在 prasertcbs Youtube 的最佳貼文
ดาวน์โหลด/git clone ไฟล์ Jupyter Notebook ที่ใช้ในคลิปได้ที่ ► https://github.com/prasertcbs/learn_numpy
เชิญสมัครเป็นสมาชิกของช่องนี้ได้ที่ ► https://www.youtube.com/subscription_center?add_user=prasertcbs
playlist สอน Numpy ► https://www.youtube.com/playlist?list=PLoTScYm9O0GFNEpzsCBEnkUwgAwOu_PWw
playlist สอน Jupyter Notebook ► https://www.youtube.com/playlist?list=PLoTScYm9O0GErrygsfQtDtBT4CloRkiDx
playlist สอน Jupyter Lab ► https://www.youtube.com/playlist?list=PLoTScYm9O0GEour5CiwfSnoutg3RyA76O
playlist สอน Pandas ► https://www.youtube.com/playlist?list=PLoTScYm9O0GGsOHPCeufxCLt-uGU5Rsuj
playlist สอน matplotlib ► https://www.youtube.com/playlist?list=PLoTScYm9O0GGRvUsTmO8MQUkIuM1thTCf
playlist สอน seaborn ► https://www.youtube.com/playlist?list=PLoTScYm9O0GGC9QvLlrQGvMYatTjnOUwR
playlist สอน Python สำหรับ data science ► https://www.youtube.com/playlist?list=PLoTScYm9O0GFVfRk_MmZt0vQXNIi36LUz
playlist สอนภาษาไพธอน Python เบื้องต้น ► https://www.youtube.com/playlist?list=PLoTScYm9O0GH4YQs9t4tf2RIYolHt_YwW
playlist สอนภาษาไพธอน Python OOP ► https://www.youtube.com/playlist?list=PLoTScYm9O0GEIZzlTKPUiOqkewkWmwadW
playlist สอน Python 3 GUI ► https://www.youtube.com/playlist?list=PLoTScYm9O0GFB1Y3cCmb9aPD5xRB1T11y
playlist สอนการใช้งานโปรแกรม R: https://www.youtube.com/playlist?list=PLoTScYm9O0GGSiUGzdWbjxIkZqEO-O6qZ
playlist สอนภาษา R เบื้องต้น ► https://www.youtube.com/playlist?list=PLoTScYm9O0GF6qjrRuZFSHdnBXD2KVIC
#prasertcbs #prasertcbs_numpy
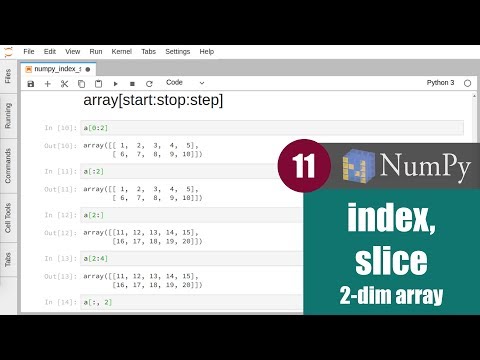
array index 在 Laravel Array Validation: Set Messages with Position/Index 的推薦與評價

Let me demonstrate how to make array validation messages more "human-friendly".Links mentioned in the video:- Multi-Language Laravel: ... ... <看更多>
array index 在 JavaScript Array indexOf and lastIndexOf: Locating an ... 的推薦與評價
To find the position of an element in an array, you use the indexOf() method. This method returns the index of the first occurrence the element that you want to ... ... <看更多>