📜 [專欄新文章] ZKP 與智能合約的開發入門
✍️ Johnson
📥 歡迎投稿: https://medium.com/taipei-ethereum-meetup #徵技術分享文 #使用心得 #教學文 #medium
這篇文章將以程式碼範例,說明 Zero Knowledge Proofs 與智能合約的結合,能夠為以太坊的生態系帶來什麼創新的應用。
本文為 Tornado Cash 研究系列的 Part 2,本系列以 tornado-core 為教材,學習開發 ZKP 的應用,另兩篇為:
Part 1:Merkle Tree in JavaScript
Part 3:Tornado Cash 實例解析
Special thanks to C.C. Liang for review and enlightenment.
近十年來最強大的密碼學科技可能就是零知識證明,或稱 zk-SNARKs (zero knowledge succinct arguments of knowledge)。
zk-SNARKs 可以將某個能得出特定結果 (output) 的計算過程 (computation),產出一個證明,而儘管計算過程可能非常耗時,這個證明卻可以快速的被驗證。
此外,零知識證明的額外特色是:你可以在不告訴對方輸入值 (input) 的情況下,證明你確實經過了某個計算過程並得到了結果。
上述來自 Vitalik’s An approximate introduction to how zk-SNARKs are possible 文章的首段,該文說是給具有 “medium level” 數學程度的人解釋 zk-SNARKs 的運作原理。(可惜我還是看不懂 QQ)
本文則是從零知識證明 (ZKP) 應用開發的角度,結合電路 (circuit) 與智能合約的程式碼來說明 ZKP 可以為既有的以太坊智能合約帶來什麼創新的突破。
基本上可以謹記兩點 ZKP 帶來的效果:
1. 擴容:鏈下計算的功能。
2. 隱私:隱藏秘密的功能。
WithoutZK.sol
首先,讓我們先來看一段沒有任何 ZKP 的智能合約:
這份合約的主軸在 process(),我們向它輸入一個秘密值 secret,經過一段計算過程後會與 answer 比對,如果驗證成功就會改寫變數 greeting 為 “answer to the ultimate question of life, the universe, and everything”。
Computation
而計算過程是一個簡單的函式:f(x) = x**2 + 6。
我們可以輕易推出秘密就是 42。
這個計算過程有很多可能的輸入值 (input) 與輸出值 (output):
f(2) = 10
f(3) = 15
f(4) = 22
…
但是能通過驗證的只有當輸出值和我們存放在合約的資料 answer 一樣時,才會驗證成功,並執行 process 的動作。
可以看到有一個 calculate 函式,說明這份合約在鏈上進行的計算,以及 process 需要輸入參數 _secret,而我們知道合約上所有交易都是公開的,所以這個 _secret 可以輕易在 etherscan 上被看到。
從這個簡單的合約中我們看到 ZKP 可以解決的兩個痛點:鏈下計算與隱藏秘密。
Circuits
接下來我們就改寫這份合約,加入 ZKP 的電路語言 circom,使用者就能用他的 secret 在鏈下進行計算後產生一個 proof,這 proof 就不會揭露有關 secret 的資訊,同時證明了當 secret 丟入 f(x) = x**2 + 6 的計算過程後會得出 1770 的結果 (output),把這個 proof 丟入 process 的參數中,經過 Verifier 的驗證即可執行 process 的內容。
有關電路 circuits 的環境配置,可以參考 ZKP Hello World,這裡我們就先跳過去,直接來看 circom 的程式碼:
template Square() { signal input in; signal output out; out <== in * in;}template Add() { signal input in; signal output out; out <== in + 6;}template Calculator() { signal private input secret; signal output out; component square = Square(); component add = Add(); square.in <== secret; add.in <== square.out; out <== add.out;}component main = Calculator();
這段就是 f(x) = x**2 + 6 在 circom 上的寫法,可能需要時間去感受一下。
ZK.sol
circom 寫好後,可以產生一個 Verifier.sol 的合約,這個合約會有一個函式 verifyProof,於是我們把上方的合約改寫成使用 ZKP 的樣子:
我們可以發現 ZK 合約少了 calculate 函式,顯然 f(x) = x**2 + 6 已經被我們寫到電路上了。
snarkjs
產生證明的程式碼以 javascript 寫成如下:
let { proof, publicSignals } = await groth16.fullProve(input, wasmPath, zkeyPath);
於是提交 proof 給合約,完成驗證,達到所謂鏈下計算的功能。
最後讓我們完整看一段 javascript 的單元測試,使用 snarkjs 來產生證明,對合約的 process 進行測試:
對合約來說, secret = 42 是完全不知情的,因此隱藏了秘密。
publicSignals
之前不太清楚 publicSignals 的用意,因此在這裡特別說明一下。
基本上在產生證明的同時,也會隨帶產生這個 circom 所有的 public 值,也就是 publicSignals,如下:
let { proof, publicSignals } = await groth16.fullProve(input, wasmPath, zkeyPath);
在我們的例子中 publicSignals 只有一個,就是 1770。
而 verifyProof 要輸入的參數除了 proof 之外,也要填入 public 值,簡單來說會是:
const isValid = verifyProof(proof, publicSignals);
問題來了,我們在設計應用邏輯時,當使用者要提交參數進行驗證的時候,publicSignals 會是由「使用者」填入嗎?或者是說,儘管是使用者填入,那它需不需要先經過檢查,才可以填入 verifyProof?
關鍵在於我們的合約上存有一筆資料:answer = 1770
回頭看合約上的 process 在進行 verifyProof 之前,必須要檢查 isAnswer(publicSignals[0]):
想想要是沒有檢查 isAnswer,這份合約會發生什麼事情?
我們的應用邏輯就會變得毫無意義,因為少了要驗證的答案,就只是完成計算 f(42) = 1770,那麼不論是 f(1) = 7 或 f(2) = 10,使用者都可以自己產生證明與結果,自己把 proof 和 publicSignals 填入 verifyProof 的參數中,都會通過驗證。
至此可以看出,ZKP 只有把「計算過程」抽離到鏈下的電路,計算後的結果仍需要與鏈上既有的資料進行比對與確認後,才能算是有效的應用 ZKP。
應用邏輯的開發
本文主要談到的是 zk-SNARKs 上層應用邏輯的開發,關於 ZKP 的底層邏輯如上述使用的 groth16 或其他如 plonk 是本文打算忽略掉的部分。
從上述的例子可以看到,即使我們努力用 circom 實作藏住 secret,但由於計算過程太過簡單,只有 f(x) = x**2+6,輕易就能從 answer 反推出我們的 secret 是 42,因此在應用邏輯的開發上,也必須注意 circom 的設計可能出了問題,導致私密訊息容易外洩,那儘管使用再強的 ZKP 底層邏輯,在應用邏輯上有漏洞,也沒辦法達到隱藏秘密的效果。
此外,在看 circom 的程式碼時,可以關注最後一個 template 的 private 與 public 值分別是什麼。以本文的 Calculator 為例,private 值有 secret,public 值有 out。
另外補充:
如果有個 signal input 但它不是 private input,就會被歸類為 public。
一個 circuit 至少會有一個 public,因為計算過程一定會有一個結果。
最後,在開發的過程中我會用 javascript 先實作計算過程,也可以順便產出 input.json,然後再用 circom 語言把計算過程實現,產生 proof 和 public 後,再去對照所有 public 值和 private 值,確認是不是符合電路計算後所要的結果,也就是比較 javascript 算出來的和 circom 算出來的一不一樣,如果不一樣就能確定程式碼是有 bug 的。
參考範例:https://github.com/chnejohnson/circom-playground
總結
本文的程式碼展現 ZKP 可以做到鏈下計算與隱藏秘密的功能,在真實專案中,可想而知電路的計算過程不會這麼單純。
會出現在真實專案中的計算像是 hash function,複雜一點會加入 Merkle Tree,或是電子簽章 EdDSA,於是就能產生更完整的應用如 Layer 2 擴容方案之一的 ZK Rollup,或是做到匿名交易的 Tornado Cash。
本文原始碼:https://github.com/chnejohnson/mini-zkp
下篇文章就來分享 Tornado Cash 是如何利用 ZKP 達成匿名交易的!
參考資料
概念介紹
Cryptography Playground
zk-SNARKs-Explainer
神奇的零知識證明!既能保守秘密,又讓別人信你!
認識零知識證明 — COSCUP 2019 | Youtube
應用零知識證明 — COSCUP 2020 | Youtube
ZK Rollup
動手實做零知識 — circom — Kimi
ZK-Rollup 开发经验分享 Part I — Fluidex
ZkRollup Tutorial
ZK Rollup & Optimistic Rollup — Kimi Wu | Medium
Circom
circom/TUTORIAL.md at master · iden3/circom · GitHub
ZKP Hello World
其他
深入瞭解 zk-SNARKs
瞭解神秘的 ZK-STARKs
zk-SNARKs和zk-STARKs解釋 | Binance Academy
[ZKP 讀書會] MACI
Semaphore
Zero-knowledge Virtual Machines, the Polaris License, and Vendor Lock-in | by Koh Wei Jie
Introduction & Evolution of ZK Ecosystem — YouTube
The Limitations of Privacy — Barry Whitehat — YouTube
Introduction to Zero Knowledge Proofs — Elena Nadolinski
ZKP 與智能合約的開發入門 was originally published in Taipei Ethereum Meetup on Medium, where people are continuing the conversation by highlighting and responding to this story.
👏 歡迎轉載分享鼓掌
同時也有5部Youtube影片,追蹤數超過7萬的網紅在地上滾的工程師 Nic,也在其Youtube影片中提到,現在學習知識的渠道越來越多,無論對於零基礎或是有經驗的工程師,想要持續成長應該看書還是看影片來的更有效率呢? 主要會和你分享我過去從新手到資深的過程中,如何持續保持進步及學習的經驗 也許這個經驗可以幫助到你,也歡迎留言和我分享你的看法 相信彼此分享不同的學習見解,能讓對於想要更精進自己程式開發...
「github tutorial」的推薦目錄:
- 關於github tutorial 在 Taipei Ethereum Meetup Facebook 的精選貼文
- 關於github tutorial 在 軟體開發學習資訊分享 Facebook 的最讚貼文
- 關於github tutorial 在 Taipei Ethereum Meetup Facebook 的最佳解答
- 關於github tutorial 在 在地上滾的工程師 Nic Youtube 的精選貼文
- 關於github tutorial 在 QVOTE Roblox and game hacks Youtube 的最佳貼文
- 關於github tutorial 在 在地上滾的工程師 Nic Youtube 的最佳解答
- 關於github tutorial 在 Hello World - GitHub Docs 的評價
- 關於github tutorial 在 An Intro to Git and GitHub for Beginners (Tutorial) - Product ... 的評價
- 關於github tutorial 在 [Git][教學] 02. 開始使用GitHub, 註冊與建立repo。 - 進度條 的評價
- 關於github tutorial 在 Git and GitHub Tutorial for Beginners - Analytics Vidhya 的評價
- 關於github tutorial 在 Git and GitHub Tutorial – Version Control for Beginners 的評價
- 關於github tutorial 在 How To Use GitHub | GitHub Tutorial For Beginners | Edureka 的評價
- 關於github tutorial 在 Github基本使用教學– 將你的NVIDIA機器學習專案都記錄起來吧! 的評價
- 關於github tutorial 在 the simple guide - no deep shit! - git 的評價
- 關於github tutorial 在 Git/Github Guide: A Minimal Tutorial - Karl Broman 的評價
- 關於github tutorial 在 What is GitHub - W3Schools 的評價
- 關於github tutorial 在 How to use GitHub and the terminal: a guide | 18F 的評價
- 關於github tutorial 在 GitHub Tutorial For Developers | How To Use GitHub 的評價
- 關於github tutorial 在 What is GitHub And How To Use It? - Simplilearn 的評價
- 關於github tutorial 在 Working with GitHub in VS Code 的評價
- 關於github tutorial 在 GitHub tutorials | Cloud Healthcare API 的評價
- 關於github tutorial 在 Introduction to GitHub in Visual Studio Code - Learn 的評價
- 關於github tutorial 在 Learn how to use Git and GitHub in a team like a pro - DEV ... 的評價
- 關於github tutorial 在 Github Tutorial: How to Make Your First GitHub Repository 的評價
- 關於github tutorial 在 A Tutorial for GitHub.pdf - ifi uzh 的評價
- 關於github tutorial 在 從0 到1 的GitHub Pages 教學手冊 的評價
- 關於github tutorial 在 Git and GitHub in a Nutshell: Definitive tutorial for beginners 的評價
- 關於github tutorial 在 GitHub Pull Request Tutorial · Thinkful Programming Guides 的評價
- 關於github tutorial 在 The easiest GitHub tutorial ever - Towards Data Science 的評價
- 關於github tutorial 在 Git & GitHub 教學手冊 - W3HexSchool - 六角學院 的評價
- 關於github tutorial 在 Introduction to Git and GitHub | Coursera 的評價
- 關於github tutorial 在 GitHub Tutorial: The Beginner's Guide | Pluralsight 的評價
- 關於github tutorial 在 Happy Git and GitHub for the useR: Let's Git started 的評價
- 關於github tutorial 在 Git Tutorial for Beginners - Learn GitHub Basic Commands 的評價
- 關於github tutorial 在 An Ultimate Guide to Git and Github - GeeksforGeeks 的評價
- 關於github tutorial 在 What Is GitHub and How Do You Use It? - Devmountain Blog 的評價
- 關於github tutorial 在 Contributing with GitHub via its interface 的評價
- 關於github tutorial 在 How to use GitHub - Simple GitHub tutorial for beginners 的評價
- 關於github tutorial 在 Git and GitHub Tutorial: Build a GitHub Portfolio and Learn ... 的評價
- 關於github tutorial 在 What is GitHub? A Simple 2021 Beginner's Guide 的評價
- 關於github tutorial 在 Tutorial on Github pages is not working 的評價
- 關於github tutorial 在 GitHub for complete beginners - The MDN project 的評價
- 關於github tutorial 在 How To Create a Pull Request on GitHub | DigitalOcean 的評價
- 關於github tutorial 在 Create a Project in SAP Web IDE and Push into GitHub 的評價
- 關於github tutorial 在 Getting started with Git and GitHub is easier than ever with ... 的評價
- 關於github tutorial 在 Host on GitHub | Hugo 的評價
- 關於github tutorial 在 Using GitHub to Share with SparkFun 的評價
- 關於github tutorial 在 Git 與Github 版本控制基本指令與操作入門教學 - TechBridge ... 的評價
- 關於github tutorial 在 The GitHub Tutorial: 10+ hacks to boost your skills - Usersnap 的評價
- 關於github tutorial 在 A Beginner's Git and GitHub Tutorial | Udacity 的評價
- 關於github tutorial 在 Tutorial Git and GitHub : Source Tree I (commit & push) - 2020 的評價
- 關於github tutorial 在 Github Desktop – A Really, Really Simple Tutorial - ClassicPress 的評價
- 關於github tutorial 在 GitHub CI/CD tutorial: Setting up continuous integration 的評價
- 關於github tutorial 在 Creating and Hosting a Personal Site on GitHub - Jonathan ... 的評價
- 關於github tutorial 在 Git and GitHub for Poets - The Coding Train 的評價
- 關於github tutorial 在 Git 101: Git and GitHub for beginners 的評價
- 關於github tutorial 在 Git for beginners: The definitive practical guide - Stack Overflow 的評價
- 關於github tutorial 在 How to create a private GitHub repository example 的評價
- 關於github tutorial 在 How To Create a Pull Request With GitHub Desktop 的評價
- 關於github tutorial 在 Step-by-step guide to contributing on GitHub - Data School 的評價
- 關於github tutorial 在 Practical Git And GitHub Tutorial In 2020 For Beginners 的評價
- 關於github tutorial 在 How To Use GitHub CLI? [Beginner's Tutorial] - Fossbytes 的評價
- 關於github tutorial 在 Git & GitHub Beginner Tutorial - Level Up Coding 的評價
- 關於github tutorial 在 Git與GitHub介紹,軟體版本控制基本教學|ALPHA Camp Blog 的評價
- 關於github tutorial 在 GitHub Actions: concepts and tutorial to get started - Padok 的評價
- 關於github tutorial 在 [1]. Git tutorials - Raywang0211/git-tutorial Wiki - GitHub Wiki ... 的評價
- 關於github tutorial 在 Everything you need to know about Git and GitHub - Android ... 的評價
- 關於github tutorial 在 Introduction to Git and GitHub for Python Developers 的評價
github tutorial 在 軟體開發學習資訊分享 Facebook 的最讚貼文
NT390 特價中
課程已於 2021 年 5 月更新
Electron 是由 Github 開發的開源框架,它允許使用 HTML/Javascript/CSS 開發同時支援 Linux/Windows/Mac 的桌面應用程式,當下許多知名桌面應用程式如 Atom,Slack 都是基於 Electron 所開發,透過這堂課,你將會跟隨著 Stephen 使用 Web 開發技術建立 4 個桌面應用程式,讓你的技術不再侷限於瀏覽器,增加不同的視野。
https://softnshare.com/electron-react-tutorial/
github tutorial 在 Taipei Ethereum Meetup Facebook 的最佳解答
📜 [專欄新文章] Optimistic Rollup 就這樣用(2)
✍️ Juin Chiu
📥 歡迎投稿: https://medium.com/taipei-ethereum-meetup #徵技術分享文 #使用心得 #教學文 #medium
ERC721 的儲值、轉移與提領
TL;DR
本文會跳過 Optimistic Rollup 的介紹而直接實際演示,關於 Optimistic Rollup 的概念與設計原理筆者將在日後另撰文說明,有興趣的讀者可以先參考下列三篇文章(由淺入深):1. OVM Deep Dive 2. (Almost) Everything you need to know about Optimistic Rollup 3. How does Optimism’s Rollup really work?
本文將演示一個 Optimism Rollup 的 ERC721 範例,程式碼在這裡。
本演示大量參考了以下範例:Optimistic Rollup Example: ERC20。
本演示所使用的 ERC721 Gateway 合約來自這個提案,目前尚未成為官方標準。
環境設置
Git
Node.js
Yarn
Docker
Docker-compose
筆者沒有碰到環境相容問題,但是建議都升到最新版本, Node.js 使用 v16.1.0 或以上版本
Optimism 服務啟動
有關 Optimisim 的所有服務,都包裝在 Optimism 這個超大專案當中了,直接使用原始碼進行組建:
$ git clone git@github.com:ethereum-optimism/optimism.git$ cd optimism$ yarn$ yarn build
組建完成後,就可以在本機啟動服務了:
$ cd ops$ docker-compose build$ docker-compose up
這個指令會啟動數個服務,包括:
L1 Ethereum Node (EVM)
L2 Ethereum Node (OVM)
Batch Submitter
Data Transport Layer
Deployer
Relayer
Verifier
Deployer 服務中的一個參數要特別注意: FRAUD_PROOF_WINDOW_SECONDS,這個就是 OPtimistic Rollup 的挑戰期,代表使用者出金(Withdraw)需等候的時長。在本篇演示中預設為 0 秒。
如果有需要重啟,記得把整個 Docker Volume 也清乾淨,例如: docker-compose down -v
Optimism 整合測試
在繼續接下來的演示之前,我們需要先確認 Optimism 是否有順利啟動,特別是 Relayer 是否運作正常,因此我們需要先進行整合測試:
$ cd optimism/integration-tests$ yarn build:integration$ yarn test:integration
確保 L1 <--> L2 Communication 相關測試通過後再繼續執行接下來的演示內容。
啟動服務及部署合約需要花費一些時間,運行一段時間(約 120 秒)之後再執行測試,如果測試結果全部皆為 Fail,可能是 Optimism 尚未啟動完成,再等待一段時間即可。
ERC721 合約部署
Optimism 啟動成功並且完成整合測試後,接下來進行 ERC721 合約的部署。筆者已將合約及部署腳本放在 optimistic-rollup-example-erc721 這個專案中:
$ git clone git@github.com:ethereum-optimism/optimistic-rollup-example-erc721.git$ cd optimistic-rollup-example-erc721$ yarn install$ yarn compile
接下來我們需要部署以下合約:
ERC721,部署於 L1
L2DepositedEERC721,部署於 L2
OVM_L1ERC721Gateway,部署於 L1
OVM_L1ERC721Gateway 只部署在 L1 上,顧名思義它就是 L1 <=> L2 的「門戶」,提供 Deposit / Withdraw 兩個基本功能,使用者必須透過這個合約來進出 L2。
雖然 OVM_L1ERC20Gateway 是 Optimistic Rollup 官方提供的合約。但是開發者也可以依需求自行設計自己的「門戶」。
OVM_L1ERC20Gateway 目前沒有 Optimism 的官方實作,本演示所使用的 ERC721 Gateway 合約來自這個提案,目前尚未成為官方標準。
接下來,我們直接用腳本進行部署:
$ node ./deploy.jsDeploying L1 ERC721...L1 ERC2721 Contract Address: 0xFD471836031dc5108809D173A067e8486B9047A3Deploying L2 ERC721...L2 ERC721 Contract Address: 0x09635F643e140090A9A8Dcd712eD6285858ceBefDeploying L1 ERC721 Gateway...L1 ERC721 Gateway Contract Address: 0xcbEAF3BDe82155F56486Fb5a1072cb8baAf547ccInitializing L2 ERC721...
ERC721 鑄造、儲值、轉移與提領
鑄造(L1)
初始狀態如下,所有帳戶皆尚未持有任何代幣:
接下來,我們將鑄造 2 個代幣以進行接下來的演示。首先,進入 ETH(L1) 的 Console:
$ npx hardhat console --network ethWelcome to Node.js v16.1.0.Type ".help" for more information.>
取得 Deployer / User 帳戶:
// In Hardhat ETH Console
> let accounts = await ethers.getSigners()
> let deployer = accounts[0]
> let user = accounts[1]
取得 ERC721 及 OVM_L1ERC721Gateway 合約物件,合約地址可以從部署訊息中取得:
// In Hardhat ETH Console
> let ERC721_abi = await artifacts.readArtifact("ExampleToken").then(c => c.abi)
> let ERC721 = new ethers.Contract("0xFD471836031dc5108809D173A067e8486B9047A3", ERC721_abi)
> let Gateway_abi = await artifacts.readArtifact("OVM_L1ERC721Gateway").then(c => c.abi)
> let Gateway = new ethers.Contract("0xcbEAF3BDe82155F56486Fb5a1072cb8baAf547cc", Gateway_abi)
鑄造兩個 ERC721 代幣:
// In Hardhat ETH Console
> await ERC721.connect(deployer).mintToken(deployer.address, "foo")
{ hash: "...", ...}
> await ERC721.connect(deployer).mintToken(deployer.address, "bar")
{ hash: "...", ...}
只有合約的 Owner(deployer) 可以進行鑄造的操作。
確認 Deployer 餘額:
> await ERC721.connect(deployer).balanceOf(deployer.address)
BigNumber { _hex: '0x02', _isBigNumber: true } // 2
確認代幣的 TokenID 與 Owner:
> await ERC721.connect(deployer).ownerOf(1)
'0xf39Fd6e51aad88F6F4ce6aB8827279cffFb92266' // deployer
> await ERC721.connect(deployer).ownerOf(2)
'0xf39Fd6e51aad88F6F4ce6aB8827279cffFb92266' // deployer
儲值(L1 => L2)
完成以上步驟後,目前的狀態如下:
接下來,授權 OVM_L1ERC721Gateway使用 TokenID 為 2 的代幣:
// In Hardhat ETH Console
> await ERC721.connect(deployer).approve("0xcbEAF3BDe82155F56486Fb5a1072cb8baAf547cc", 2)
{ hash: "...", ...}
在 OVM_L1ERC721Gateway 合約呼叫 Deposit,儲值 TokenID 為 2 的代幣:
// In Hardhat ETH Console
> await Gateway.connect(deployer).deposit(2)
{ hash: "...", ...}
我們可以到 Optimism (L2) 的 Console 確認入金是否成功:
$ npx hardhat console --network optimismWelcome to Node.js v16.1.0.Type ".help" for more information.>
取得 Deployer / User 帳戶:
// In Hardhat Optimism Console
> let accounts = await ethers.getSigners()
> let deployer = accounts[0]
> let user = accounts[1]
取得 L2DepositedERC721 合約物件,合約地址可以從部署訊息中取得:
// In Hardhat Optimism Console
> let L2ERC721_abi = await artifacts.readArtifact("OVM_L2DepositedERC721").then(c => c.abi)
> let L2DepositedERC721 = new ethers.Contract("0x09635F643e140090A9A8Dcd712eD6285858ceBef", L2ERC721_abi)
確認入金是否成功:
// In Hardhat Optimism Console
> await L2DepositedERC721.connect(deployer).balanceOf(deployer.address)
BigNumber { _hex: '0x01', _isBigNumber: true } // 1
> await L2DepositedERC721.connect(deployer).ownerOf(2)
'0xf39Fd6e51aad88F6F4ce6aB8827279cffFb92266' // deployer
ERC721 轉移(L2 <=> L2)
完成以上步驟後,目前的狀態如下:
接下來,我們在 L2 從 Deployer 轉移代幣給 User:
// In Hardhat Optimism Console
> await L2DepositedERC721.connect(user).balanceOf(user.address)
BigNumber { _hex: '0x00', _isBigNumber: true } // 0
> await L2DepositedERC721.connect(deployer).transferFrom(depoyer.address, user.address, 2)
{ hash: "..." ...}
> await L2DepositedERC721.connect(user).balanceOf(user.address)
BigNumber { _hex: '0x01', _isBigNumber: true } // 1
> await L2DepositedERC721.connect(user).ownerOf(2)
'0x70997970C51812dc3A010C7d01b50e0d17dc79C8' // user
ERC721 提領(L2 => L1)
完成以上步驟後,目前的狀態如下:
接下來,我們用 User 帳戶提領資金,在 L2DepositedERC721 合約呼叫 Withdraw:
// In Hardhat Optimism Console
> await L2DepositedERC721.connect(user).withdraw(2)
{ hash: "..." ...}
> await L2DepositedERC721.connect(user).balanceOf(user.address)
BigNumber { _hex: '0x00', _isBigNumber: true }
最後,檢查在 L1 是否提領成功:
// In Hardhat ETH Console
> await ERC721.connect(user).balanceOf(user.address)
BigNumber { _hex: '0x01', _isBigNumber: true } // 1
> await ERC721.connect(deployer).balanceOf(deployer.address)
BigNumber { _hex: '0x01', _isBigNumber: true } // 1
> await ERC721.connect(user).ownerOf(2)
'0x70997970C51812dc3A010C7d01b50e0d17dc79C8' // user
由於挑戰期為 0 秒,因此提領幾乎無需等待時間,頂多只需數秒鐘
做完上述所有操作,最終狀態應該如下:
總結
本文演示了:
Optimistic Rollup 相關服務的本機部署
ERC721 L1 => L2 的儲值(Deposit)
ERC721 L2 帳戶之間轉移(Transfer)
ERC721 L2 => L1 的提領(Withdraw)
筆者未來將繼續擴充此系列的教學內容,例如支援其他標準的合約如 ERC1155,以及如何運行 Optimistic Rollup 生態系中最重要的驗證者(Verifier),敬請期待。
參考資料
OVM Deep Dive
(Almost) Everything you need to know about Optimistic Rollup
How does Optimism’s Rollup really work?
Optimistic Rollup Official Documentation
Ethers Documentation (v5)
Optimistic Rollup Example: ERC20(Github)
Optimism (Github)
optimism-tutorial (Github)
l1-l2-deposit-withdrawal (Github)
Proof-of-concept ERC721 Bridge Implementation (Github)
Optimistic Rollup 就這樣用(2) was originally published in Taipei Ethereum Meetup on Medium, where people are continuing the conversation by highlighting and responding to this story.
👏 歡迎轉載分享鼓掌
github tutorial 在 在地上滾的工程師 Nic Youtube 的精選貼文
現在學習知識的渠道越來越多,無論對於零基礎或是有經驗的工程師,想要持續成長應該看書還是看影片來的更有效率呢?
主要會和你分享我過去從新手到資深的過程中,如何持續保持進步及學習的經驗
也許這個經驗可以幫助到你,也歡迎留言和我分享你的看法
相信彼此分享不同的學習見解,能讓對於想要更精進自己程式開發功力的人有很大的幫助
===章節===
00:00 哪一個有效律?
00:36 寫程式如同寫作
05:14 書是最便宜的資源
10:14 折扣碼操作示範
===蝦皮購書折扣碼===
折扣碼:FLAGNIC36
時間:2021-03-29 ~ 2021-06-29
折扣碼:FLAGNIC79
時間:2021-06-30 ~ 2021-09-30
折扣碼: FLAGNIC11
時間:2021-10-01~ 2021-12-31
===前陣子在看的推薦書單===
(零基礎)
- 白話演算法!培養程式設計的邏輯思考
- Python 刷提鍛鍊班
(中高階)
- 設計模式之禪(第2版)
- 無瑕的程式碼-整潔的軟體設計與架構篇
- 單元測試的藝術
- 演算法之美:隱藏在資料結構背後的原理(C++版)
- Kent Beck的實作模式
(Ruby)
- Writing Efficient Ruby Code
(成長思考)
- 圖解.實戰 麥肯錫式的思考框架:讓大腦置入邏輯,就能讓90%的困難都有解!
- 師父:那些我在課堂外學會的本事
- 高勝算決策:如何在面對決定時,降低失誤,每次出手成功率都比對手高?
- 窮查理的普通常識
- 懶人圖解簡報術:把複雜知識變成一看就秒懂的圖解懶人包
- 寫作,是最好的自我投資
喜歡影片的話!可以幫忙點個喜歡以及分享、訂閱唷!😘
━━━━━━━━━━━━━━━━
🎬 觀看我的生活廢片頻道: https://bit.ly/2Ldfp1B
⭐ instagram (生活日常): https://www.instagram.com/niclin_tw/
⭐ Facebook (資訊分享): https://www.facebook.com/niclin.dev
⭐ Blog (技術筆記): https://blog.niclin.tw
⭐ Linkedin (個人履歷): https://www.linkedin.com/in/nic-lin
⭐ 蝦皮賣場: https://shopee.tw/bboyceo
⭐ Github: https://github.com/niclin
⭐ Podcast: https://anchor.fm/niclin
━━━━━━━━━━━━━━━━
✉️ 合作邀約信箱: niclin0226@gmail.com
#寫程式 #前端 #後端
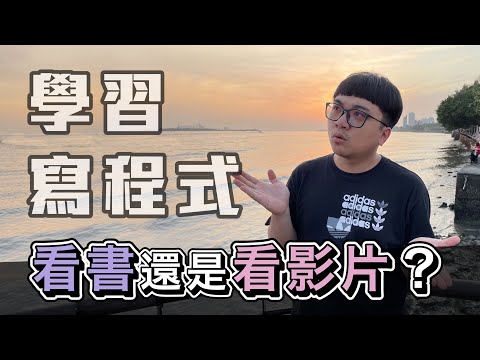
github tutorial 在 QVOTE Roblox and game hacks Youtube 的最佳貼文
✅DOWNLOAD: https://bit.ly/3vVpFxY
TAGS:
fivem hack menu,
fivem hack money,
fivem hacks 2021,
fivem hack free,
fivem hacker,
fivem hacking script,
fivem hack menu free,
fivem hack cheat engine,
fivem hack 2021,
fivem hack menu free download,
fivem hack free money,
fivem hack aimbot,
fivem hack menu admin,
fivem hack britain,
fivem hack bypass,
fivem hack buy,
bhutan fivem hack,
fivem bank hack,
best hack fivem,
fivem hack brasil,
como baixar hack fivem,
fivem hack car,
fivem hack client,
fivem weapon hack cheat engine,
fivem crosshair hack,
fivem casino hack,
#fivem #hack #menu
cara hack fivem,
fivem cash hack,
fivem hack download,
fivem hack discord,
fivem hack dll,
fivem hack download free,
fivem hack dansk,
fivem money hack free download,
fivem drug locations hack,
fivem dirty money hack,
fivem money hack cheat engine,
hack fivem cheat engine,
fivem esx hack,
fivem esx money hack,
fivem esp hack,
fivem external hack,
fivem hack externo,
fivem hack free download,
fivem hack free 2020,
fivem hack free romania,
gta fivem hack free,
fivem hack guns,
gta v fivem hack,
gta v fivem hack 2020,
gta 5 fivem hack 2020,
gta v fivem hack money 2019,
fivem hack gratis,
hack para fivem rp gratis,
how to hack fivem,
how to hack fivem servers,
how to hack fivem money,
how to hack fivem mod menu,
how to hack fivem 2020,
how to hack fivem cheat engine,
huong dan hack fivem,
fivem hack injector,
fivem hack indonesia,
fivem hack lynx,
fivem hack lua,
fivem money hack lua,
fivem legacy hack,
fivem lua hack free,
fivem hack money free,
fivem hack mod menu,
fivem hack menu 2020,
fivem hack menu free 2020,
new fivem hack,
hack no fivem,
hack on fivem,
fivem hack ops,
popstar hack fivem,
hack para fivem,
hack pentru fivem,
hack para fivem 2020,
hack para fivem rp,
hack para fivem gratis,
hack free para fivem,
fivem hack romania,
fivem rp money hack,
fivem rp hack,
fivem roleplay hack,
fivem hack red engine,
gta rp fivem hack,
fivem hack script,
fivem server money hack,
fivem slot machine hack,
fivem hack shaniu,
fivem hack stream,
fivem hack tiago,
fivem hack troll,
fivem hack tutorial,
transport tycoon fivem hack,
fivem hack unban,
fivem money hack undetected,
usando hack fivem,
vmenu fivem hack,
hack gta v fivem free,
hack gta v fivem 2019 free,
fivem vrp hack,
fivem vehicle hack,
gta v fivem hack money,
gta v fivem hacks,
fivem hack weapon,
wall hack fivem,
hack whitelist fivem,
fivem 저 hack,
fivem hack 2020 free,
fivem money hack 2020,
fivem money hack 2019 free,
fivem money hack 2019,
gta 5 fivem hack,
hack para gta 5 fivem,
gta 5 fivem hack free,
gta 5 fivem hacks,
gta 5 fivem hacker
fivem lua executor
fivem lua executor 2020
fivem lua executor free download
fivem lua executor 2019
fivem lua executor github
fivem lua executor source
fivem lua executor buy
fivem lua executor commands
fivem lua executor paid
fivem lua executor discord
fivem lua executor scripts
fivem lua executor cracked
fivem lua executor unknowncheats
fivem lua executor free
fivem scripthook bypass and lua executor
fivem lua executor codes
fivem lua executor source code
fivem lua executor download
fivem lua executor error
fivem lua executor example
fivem lua executor event
gta 5 fivem lua executor
fivem lua executor host
fivem lua executor id
fivem lua executor install
fivem lua executor job
fivem lua executor join
fivem lua executor jar
fivem lua executor key
fivem lua executor keys
fivem lua executor location
fivem lua executor list
fivem lua executor mode
fivem lua executor missing
fivem lua executor mac
fivem lua executor name
fivem lua executor not working
fivem lua executor not found
fivem lua executor options
fivem lua executor online
fivem lua executor quest
fivem lua executor quests
fivem lua executor questions
fivem lua executor query
fivem lua executor template
fivem lua executor tutorial
fivem lua executor version
fivem lua executor reset
fivem lua executor roblox
fivem lua executor xp
fivem lua executor youtube
how to make a lua executor fivem
lua executor fivem buy
fivem lua executor zip
fivem lua executor zone
fivem lua executor zero
lua executor fivem download
free fivem lua executor
unknowncheats fivem lua executor

github tutorial 在 在地上滾的工程師 Nic Youtube 的最佳解答
軟體工程師在進入職場後,前 3 年是想法塑形以及定義價值觀的最佳時機,往往在初期沒建立好的觀念和實力,到了後面更是被日新月異的技術以及更強更年輕的新血注入給淹沒。
要如何保持高速成長及不斷學習,是工程師生涯得不斷思考的問題,而這部影片將會和你分享,我如何用日本劍道的三字心訣,分析我自己生涯的前三年在做的學習,並一步步判斷自己成長學習的階段及改善。
章節:
00:00 有投票一定要來看
01:02 學習三階段
03:17 刻意練習的第一年
07:15 成長衝刺的二至三年
09:54 三年以後呢?
喜歡影片的話!可以幫忙點個喜歡以及分享、訂閱唷!😘
━━━━━━━━━━━━━━━━
⭐ 蝦皮賣場: https://shopee.tw/bboyceo
⭐ instagram (生活日常): https://www.instagram.com/niclin_tw/
⭐ Facebook (資訊分享): https://www.facebook.com/niclin.dev
⭐ Blog (技術筆記): https://blog.niclin.tw
⭐ Linkedin (個人履歷): https://www.linkedin.com/in/nic-lin
⭐ Github: https://github.com/niclin
⭐ Podcast: https://anchor.fm/niclin
━━━━━━━━━━━━━━━━
🌟 任何問題或合作邀約信箱: niclin0226@gmail.com
#前端 #後端 #工程師 #守破離
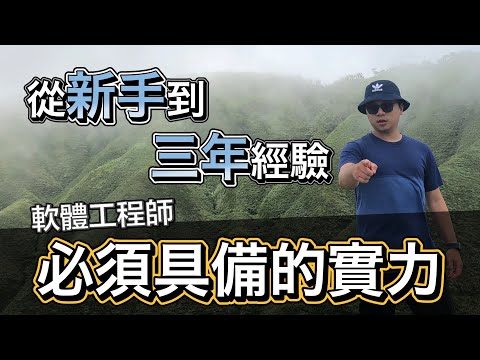
github tutorial 在 An Intro to Git and GitHub for Beginners (Tutorial) - Product ... 的推薦與評價
An Intro to Git and GitHub for Beginners (Tutorial) · Step 0: Install git and create a GitHub account · Step 1: Create a local git repository · Step 2: Add a new ... ... <看更多>
github tutorial 在 [Git][教學] 02. 開始使用GitHub, 註冊與建立repo。 - 進度條 的推薦與評價
[Git][教學] 02. 開始使用GitHub, 註冊與建立repo。 介紹如何申請Github帳號,以及如何利用它與本地端的電腦連接。 作者: 進度條編輯群 更新 ... ... <看更多>
github tutorial 在 Hello World - GitHub Docs 的推薦與評價
This tutorial teaches you GitHub essentials like repositories, branches, commits, and pull requests. You'll create your own Hello World repository and learn ... ... <看更多>
相關內容